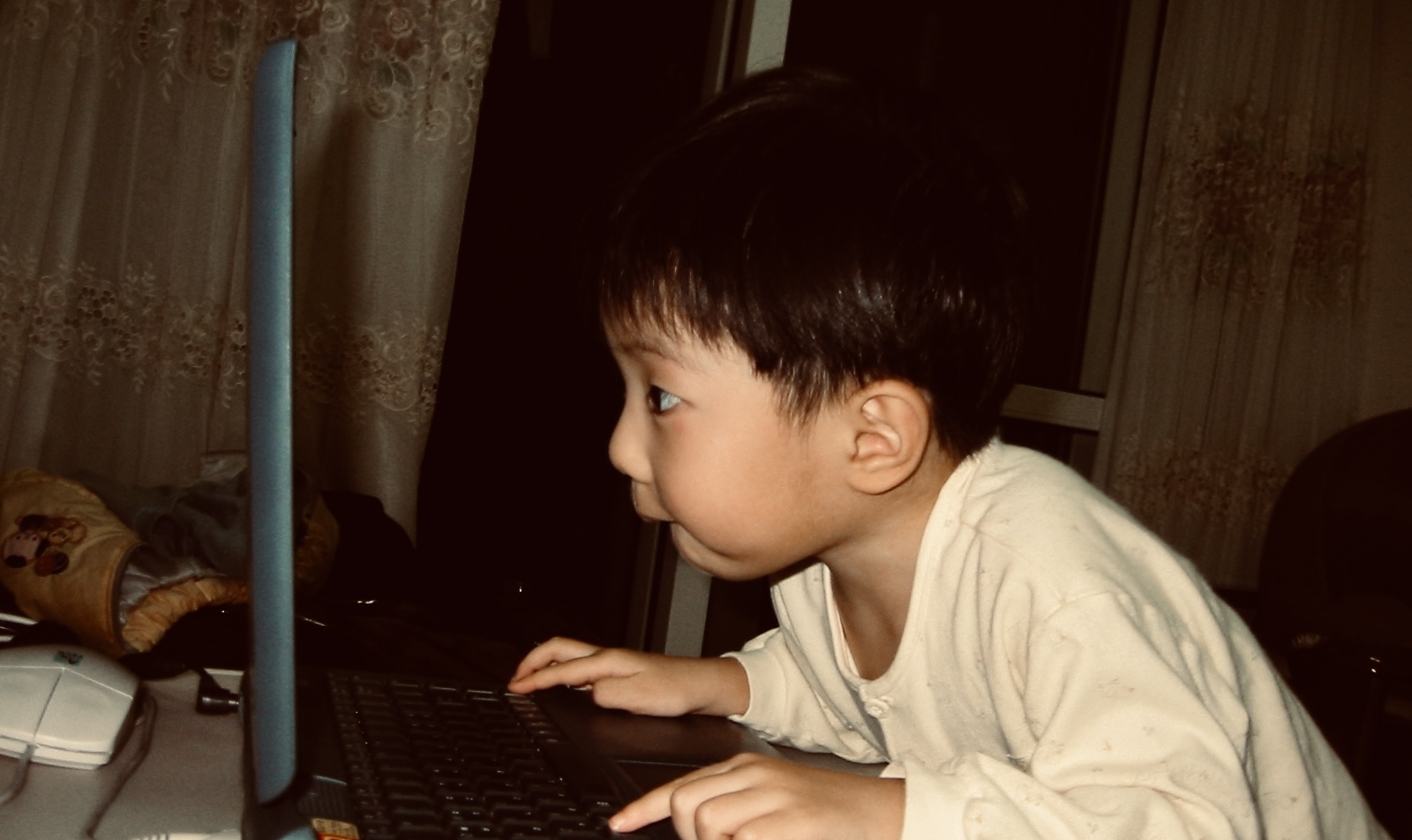
이성열 yeolyi
배우고 경험한 것들을 다듬어 여기에 공유해요.
카카오에서 프론트엔드 개발을 합니다.
서울대학교에서 컴퓨터공학을 복수전공합니다.
게시물
자바스크립트 공부 기록
값
값
자바스크립트에 있는 값들의 종류와 형변환 과정을 공부했습니다.
연
연산자
값들을 연산자로 조합해 새로운 값을 만드는 방법을 공부했습니다.
구
구문
여러 구문을 모아 프로그램을 만드는 방법을 공부했습니다.
객
객체
자바스크립트의 가장 중요한 주제인 객체를 공부했습니다.
배
배열
다른 언어들과 미묘하게 다른 자바스크립트의 배열을 공부했습니다.
함
함수
맥락에 따라 다르게 동작하는 자바스크립트의 함수와 this 키워드를 공부했습니다.
클
클래스
클래스의 성질이 자바스크립트에서 어떻게 구현되는지 공부했습니다.
모
모듈
모듈이 왜 필요한지, 자바스크립트에 어떤 종류의 모듈 시스템이 있는지 공부했습니다.
라
라이브러리
자바스크립트 표준 라이브러리에 어떤 것들이 있는지 공부했습니다.
이
이터레이터
데이터에 순서대로 접근하는 과정을 어떻게 추상화했는지 공부했습니다.
비
비동기 프로그래밍
자바스크립트가 비동기 작업을 어떻게 표현하며 어떻게 처리하도록하는지 공부했습니다.
메
메타 프로그래밍
코드를 수정하는 코드인 메타 프로그래밍에 관련된 API들을 공부했습니다.
Web API 공부 기록
Web API 기초
브라우저에서 JS가 어떤 과정으로 실행되는지 배웠습니다
이벤트
브라우저에서 이벤트가 어떻게 발생되고 전파되는지 공부했습니다.
Document 조작
JS로 웹페이지의 내용을 바꾸는 방법을 공부했습니다.
CSS 조작
JS로 CSS 스타일을 바꾸는 방법을 공부했습니다
좌표와 스크롤
요소의 크기와 위치, 스크롤을 알고 조작하는 법을 공부했습니다.
컴포넌트
네이티브로 컴포넌트를 구현하는 방법을 공부했습니다
SVG
해상도 상관없이 깔끔하게 렌더링되는 이미지 포맷을 공부했습니다
Navigation
히스토리 관련된 API들과 함께 pushState()로 상태를 관리하는 방법을 공부했습니다.
Network
자바스크립트로 직접 네트워크 요청을 보내는 방법을 공부했습니다.
Storage
브라우저상에 데이터를 저장하는 다양한 방법을 공부했습니다.
Worker
별개의 스레드로 JS 코드를 실행시키는 방법을 공부했습니다.